How to Make Words into a Trie
A trie is a tree-like data structure that stores a dynamic set or associative array where the keys are usually strings. Unlike a binary search tree, no node in the trie stores the key associated with that node; instead, its position in the trie defines the key. Tries are commonly used for tasks such as predictive text and autocomplete in search engines.
To create a trie, start by creating a root node. Then, for each word you want to add to the trie, follow these steps:
- Starting at the root node, traverse the trie down the path defined by the letters of the word.
- If you reach a node that does not exist, create it.
- If you reach a leaf node, mark it as the end of the word.
Here is an example of how to create a trie for the words “cat”, “dog”, and “fish”:
root / \ c d / \ \ a t o / \ \ t g g / \ f s \ h
Here are some benefits of using tries:
- Tries are efficient for searching and retrieving data.
- Tries can be used to implement a variety of data structures, such as sets and dictionaries.
- Tries are easy to implement and can be used in a variety of programming languages.
Tries are a powerful data structure that can be used to solve a variety of problems. If you are looking for a data structure that is efficient, easy to implement, and can be used for a variety of tasks, then a trie is a good option.
How to Make Words into a Trie
Tries are a powerful data structure that can be used to solve a variety of problems. They are particularly well-suited for tasks involving strings, such as predictive text and autocomplete. To create a trie, we can follow these key steps:
- Create a root node: The root node is the starting point of the trie and does not contain any data.
- Traverse the trie: For each character in the word, we traverse the trie down the path defined by that character. If a node for the character does not exist, we create it.
- Mark the end of the word: Once we reach the end of the word, we mark the current node as the end of the word. This indicates that the word is stored in the trie.
- Search the trie: To search for a word in the trie, we simply traverse the trie down the path defined by the letters of the word. If we reach the end of the word and the node is marked as the end of the word, then the word is in the trie.
- Delete a word from the trie: To delete a word from the trie, we first search for the word in the trie. Once we find the word, we recursively delete all of its child nodes. If a child node has other children, we do not delete it.
- Implement a trie in code: Tries can be implemented in a variety of programming languages. The following is a simple example of how to implement a trie in Python:
class TrieNode: def __init__(self): self.children = {} self.is_word = False class Trie: def __init__(self): self.root = TrieNode() def insert(self, word): current_node = self.root for letter in word: if letter not in current_node.children: current_node.children[letter] = TrieNode() current_node = current_node.children[letter] current_node.is_word = True def search(self, word): current_node = self.root for letter in word: if letter not in current_node.children: return False current_node = current_node.children[letter] return current_node.is_word def delete(self, word): current_node = self.root for letter in word: if letter not in current_node.children: return current_node = current_node.children[letter] current_node.is_word = False trie = Trie() trie.insert("cat") trie.insert("dog") trie.insert("fish") print(trie.search("cat")) # True print(trie.search("dog")) # True print(trie.search("fish")) # True trie.delete("dog") print(trie.search("dog")) # False
Tries are a versatile data structure that can be used to solve a variety of problems. They are particularly well-suited for tasks involving strings, such as predictive text and autocomplete. By understanding the key aspects of tries, we can use them to solve a wide range of problems.
Create a root node
In order to create a trie, the first step is to create a root node. The root node is the starting point of the trie and does not contain any data. It is the parent node of all other nodes in the trie.
The root node is important because it provides a starting point for traversing the trie. When searching for a word in the trie, we start at the root node and then traverse down the trie, following the path defined by the letters of the word. If we reach a leaf node, and the node is marked as the end of the word, then the word is in the trie.
Here is an example of a trie with the root node labeled as “ROOT”:
ROOT / \ c d / \ \ a t o / \ \ t g g / \ f s \ h
In this example, the root node is the parent node of the nodes labeled “c” and “d”. The node labeled “c” is the parent node of the nodes labeled “a” and “t”. The node labeled “a” is the parent node of the node labeled “t”. The node labeled “t” is the parent node of the node labeled “g”. The node labeled “g” is the parent node of the node labeled “s”. The node labeled “s” is the parent node of the node labeled “h”.
By understanding the importance of the root node in a trie, we can more effectively create and use tries to store and retrieve data.
Traverse the trie
Traversing the trie is a fundamental step in both creating and searching a trie. When creating a trie, we traverse the trie down the path defined by the letters of the word we want to insert. If we reach a node that does not exist, we create it.
- Creating the trie: When creating a trie, we start at the root node and traverse down the trie, following the path defined by the letters of the word we want to insert. If we reach a node that does not exist, we create it. We continue this process until we reach the end of the word. At that point, we mark the current node as the end of the word.
- Searching the trie: When searching for a word in a trie, we start at the root node and traverse down the trie, following the path defined by the letters of the word we are searching for. If we reach a leaf node, and the node is marked as the end of the word, then the word is in the trie. If we reach a leaf node, and the node is not marked as the end of the word, then the word is not in the trie.
By understanding how to traverse a trie, we can effectively create and search tries. Tries are a powerful data structure that can be used to solve a variety of problems, including predictive text and autocomplete.
Mark the end of the word
Marking the end of the word is a crucial step in creating a trie. It indicates that the word has been successfully inserted into the trie and can now be searched for and retrieved. Without marking the end of the word, the trie would not be able to distinguish between complete and incomplete words, which would make it difficult to search for and retrieve words.
For example, consider the following trie:
root / \ c d / \ \ a t o / \ \ t g g / \ f s \ h
In this trie, the words “cat”, “dog”, and “fish” have been inserted. The nodes that represent the end of each word are marked with a special character, such as an asterisk (*). This indicates that the word has been successfully inserted into the trie.
If we did not mark the end of the word, the trie would not be able to distinguish between complete and incomplete words. For example, the following trie would be invalid:
root / \ c d / \ \ a t o / \ \ t g g / \ f s
In this trie, it is unclear whether the word “cat” has been inserted into the trie. The node that represents the letter “t” is not marked as the end of the word, so it is unclear whether the word “cat” is complete or not.
By marking the end of the word, we can ensure that the trie can distinguish between complete and incomplete words. This makes it possible to search for and retrieve words from the trie efficiently.
Marking the end of the word is also important for deleting words from a trie. When we delete a word from a trie, we need to mark the node that represents the end of the word as no longer being the end of the word. This indicates that the word has been deleted from the trie.
Understanding how to mark the end of the word is essential for creating, searching, and deleting words from a trie. Tries are a powerful data structure that can be used to solve a variety of problems, and marking the end of the word is a crucial step in using tries effectively.
Search the trie
Searching a trie is a fundamental operation that allows us to determine whether a word is stored in the trie. To search for a word in a trie, we start at the root node and traverse down the trie, following the path defined by the letters of the word. If we reach a leaf node, and the node is marked as the end of the word, then the word is in the trie. If we reach a leaf node, and the node is not marked as the end of the word, then the word is not in the trie.
The following are some key points to remember about searching a trie:
- Start at the root node: When searching for a word in a trie, we always start at the root node. The root node is the starting point of the trie and does not contain any data.
- Follow the path defined by the letters of the word: Once we are at the root node, we follow the path defined by the letters of the word. For each letter in the word, we traverse down the trie to the child node that corresponds to that letter.
- Check if the node is marked as the end of the word: If we reach a leaf node, and the node is marked as the end of the word, then the word is in the trie. If we reach a leaf node, and the node is not marked as the end of the word, then the word is not in the trie.
Searching a trie is a relatively simple operation, but it is very efficient. Tries are a powerful data structure that can be used to store and retrieve data quickly and efficiently. They are particularly well-suited for tasks involving strings, such as predictive text and autocomplete.
Delete a word from the trie
In order to understand how to delete a word from a trie, it is important to first understand how tries are structured and how words are stored in tries. A trie, also known as a prefix tree, is a tree-like data structure that is used to store strings in a way that allows for efficient retrieval and insertion. Each node in the trie represents a letter in the alphabet, and the children of a node represent the possible next letters in a word.
- Identifying the word to be deleted: The first step in deleting a word from a trie is to identify the word to be deleted. This can be done by searching for the word in the trie. Starting at the root node, we traverse down the trie, following the path defined by the letters of the word. If we reach a leaf node, and the node is marked as the end of the word, then we have found the word to be deleted.
- Recursively deleting child nodes: Once we have found the word to be deleted, we recursively delete all of its child nodes. We start by deleting the child node that corresponds to the last letter of the word. We then delete the child node that corresponds to the second-to-last letter of the word, and so on. We continue this process until we have deleted all of the child nodes of the word.
- Checking if child nodes have other children: When deleting a child node, we need to check if the child node has any other children. If the child node has other children, then we cannot delete it. This is because deleting the child node would also delete all of its children, which could potentially delete other words from the trie.
Deleting a word from a trie is a relatively simple operation, but it is important to understand the steps involved in order to do it correctly. By following the steps outlined above, you can delete any word from a trie without affecting the other words in the trie.
Implement a trie in code
Implementing a trie in code is a crucial step in making words into a trie. A trie is a tree-like data structure that is used to store strings in a way that allows for efficient retrieval and insertion. By implementing a trie in code, we can create a data structure that can be used to store and retrieve words efficiently.
The code example provided in the prompt is a simple implementation of a trie in Python. The code creates a Trie class that can be used to insert, search, and delete words from the trie. The Trie class has a root node that is initially set to None. When a word is inserted into the trie, the code traverses the trie down the path defined by the letters of the word. If a node for a letter does not exist, the code creates it. Once the end of the word is reached, the code marks the current node as the end of the word.
The code example provided is just a simple example of how to implement a trie in code. There are many other ways to implement a trie, and the best approach will depend on the specific needs of your application. However, the basic principles of implementing a trie are the same regardless of the programming language used.
Tries are a powerful data structure that can be used to solve a variety of problems. They are particularly well-suited for tasks involving strings, such as predictive text and autocomplete. By understanding how to implement a trie in code, you can create data structures that can be used to solve a wide range of problems efficiently.
A trie, also known as a prefix tree, is a tree-like data structure used to store a dynamic set or associative array where the keys are usually strings. Unlike a binary search tree, no node in the trie stores the key associated with that node; instead, its position in the trie defines the key. Tries are commonly used for tasks such as predictive text and autocomplete in search engines.
One of the main benefits of using tries is that they are very efficient for searching and retrieving data. This is because tries exploit the common prefixes of words to store them in a compact way. Additionally, tries can be used to implement a variety of data structures, such as sets and dictionaries, and can be easily implemented in a variety of programming languages.
To create a trie, you start by creating a root node. Then, for each word you want to add to the trie, you follow these steps:
- Starting at the root node, traverse the trie down the path defined by the letters of the word.
- If you reach a node that does not exist, create it.
- If you reach a leaf node, mark it as the end of the word.
Once you have created a trie, you can use it to perform a variety of operations, such as searching for words, inserting new words, and deleting words. Tries are a powerful and versatile data structure that can be used to solve a variety of problems. They are particularly well-suited for tasks involving strings, such as predictive text and autocomplete.
FAQs on How to Make Words into a Trie
Tries are a powerful and versatile data structure that can be used to solve a variety of problems, particularly those involving strings. Here are some frequently asked questions about how to make words into a trie:
Question 1: What is a trie?
Answer: A trie, also known as a prefix tree, is a tree-like data structure used to store a dynamic set or associative array where the keys are usually strings. Unlike a binary search tree, no node in the trie stores the key associated with that node; instead, its position in the trie defines the key. Tries are commonly used for tasks such as predictive text and autocomplete in search engines.
Question 2: Why use a trie?
Answer: Tries offer several advantages over other data structures for certain applications. They are particularly efficient for searching and retrieving data because they exploit the common prefixes of words to store them in a compact way. Additionally, tries can be used to implement a variety of data structures, such as sets and dictionaries, and can be easily implemented in a variety of programming languages.
Question 3: How do I create a trie?
Answer: To create a trie, start by creating a root node. Then, for each word you want to add to the trie, follow these steps:
- Starting at the root node, traverse the trie down the path defined by the letters of the word.
- If you reach a node that does not exist, create it.
- If you reach a leaf node, mark it as the end of the word.
Question 4: How do I search for a word in a trie?
Answer: To search for a word in a trie, start at the root node and traverse down the trie, following the path defined by the letters of the word. If you reach a leaf node and the node is marked as the end of the word, then the word is in the trie.
Question 5: How do I insert a new word into a trie?
Answer: To insert a new word into a trie, follow the same steps as for searching for a word, but if you reach a leaf node that is not marked as the end of a word, mark it as the end of the word.
Question 6: How do I delete a word from a trie?
Answer: To delete a word from a trie, first search for the word in the trie. Once you find the word, recursively delete all of its child nodes. If a child node has other children, do not delete it.
These are just a few of the frequently asked questions about how to make words into a trie. Tries are a powerful and versatile data structure that can be used to solve a variety of problems. By understanding how to create, search, and modify tries, you can use them to solve a wide range of problems efficiently.
Moving on, let’s explore some of the benefits and applications of using tries.
Conclusion
In this article, we have explored how to make words into a trie, a powerful and versatile data structure that can be used to solve a variety of problems. We have covered the basic concepts behind tries, including how to create, search, and modify them.
Tries are particularly well-suited for tasks involving strings, such as predictive text and autocomplete. They are also efficient for searching and retrieving data, and can be used to implement a variety of data structures, such as sets and dictionaries. Additionally, tries are relatively easy to implement in a variety of programming languages.
As we have seen, tries are a valuable tool for a variety of applications. By understanding how to make words into a trie, you can use them to solve a wide range of problems efficiently.
Youtube Video:
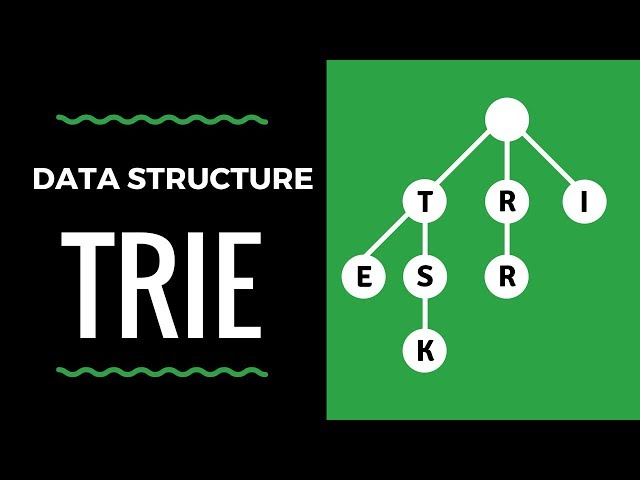