How to Make a Voxel Game in C
Voxel games are a popular genre of video games that use voxels, or three-dimensional cubes, to create their worlds. They are often used to create games with a retro or blocky aesthetic, such as Minecraft and Roblox. Creating a voxel game in C can be a challenging but rewarding experience, and this guide will provide you with the steps and guidelines you need to get started.
Steps to Create a Voxel Game in C:
- Create a new C project.
- Set up your graphics library. You will need a graphics library to render your game’s world. There are many different graphics libraries available, such as OpenGL, DirectX, and SDL.
- Create a world generator. The world generator will create the terrain for your game. You can use a variety of algorithms to generate your terrain, such as perlin noise or fractal algorithms.
- Create a player character. The player character is the character that the player controls in the game. You will need to create a model for your player character, as well as code to control its movement and interactions.
- Add gameplay elements. Once you have a basic game world and player character, you can start adding gameplay elements, such as enemies, items, and puzzles.
- Test and debug your game. Once you have added all of the desired gameplay elements, you will need to test and debug your game to ensure that it is working properly.
Benefits of Creating a Voxel Game in C:
- C is a powerful and efficient programming language. This makes it a good choice for creating voxel games, which can be computationally intensive.
- C gives you full control over the game’s engine. This allows you to customize the game to your liking and create unique experiences.
- There are many resources available online to help you create voxel games in C. This includes tutorials, forums, and code libraries.
Creating a voxel game in C can be a challenging but rewarding experience. By following the steps and guidelines outlined in this guide, you can create your own unique and immersive voxel game.
Essential Aspects of Creating a Voxel Game in C
Creating a voxel game in C involves mastering various key aspects. Here are eight essential aspects to consider:
- Graphics Library: Choose a suitable graphics library (e.g., OpenGL, DirectX) for rendering the game’s world.
- World Generation: Develop algorithms (e.g., perlin noise) to generate the game’s terrain.
- Player Character: Create a model and control mechanisms for the player character.
- Gameplay Elements: Design and implement gameplay elements like enemies, items, and puzzles.
- Physics Engine: Integrate a physics engine to handle interactions between objects.
- Lighting and Shading: Implement lighting and shading techniques to enhance the game’s visuals.
- Optimization: Optimize the game’s performance through techniques like culling and level of detail.
- Networking: Enable multiplayer functionality by implementing networking protocols.
These aspects are interconnected and require careful consideration. For instance, the choice of graphics library impacts the game’s visual quality and performance. World generation algorithms determine the game’s environment and gameplay possibilities. By understanding and mastering these aspects, developers can create engaging and immersive voxel games in C.
Graphics Library
The choice of graphics library is crucial in voxel game development, as it directly affects the game’s visual quality, performance, and overall aesthetics. A well-chosen graphics library can enhance the immersion and enjoyment of the gameplay experience.
OpenGL and DirectX are two popular graphics libraries widely used in game development. OpenGL is an industry-standard, cross-platform library that provides a comprehensive set of features for 3D graphics rendering. DirectX, on the other hand, is a Microsoft-developed library specifically optimized for Windows systems, offering high performance and compatibility with DirectX-compatible hardware.
When selecting a graphics library for a voxel game in C, factors such as the target platform, desired visual effects, and performance requirements should be considered. OpenGL is a suitable choice for cross-platform development and offers a wide range of features, while DirectX provides optimized performance on Windows systems. Understanding the capabilities and limitations of each library is essential for making an informed decision.
By choosing an appropriate graphics library that aligns with the game’s requirements, developers can create visually stunning and performant voxel games that captivate players.
World Generation
In the context of voxel games, world generation plays a pivotal role in shaping the game’s environment and gameplay possibilities. By developing algorithms, such as perlin noise, developers can create dynamic and immersive worlds that enhance the player’s experience.
- Terrain Generation: Perlin noise is a widely used algorithm for generating realistic terrain in voxel games. It produces natural-looking landscapes with smooth transitions and varying elevations, creating a diverse and visually appealing environment for players to explore.
- Procedural Generation: World generation algorithms like perlin noise enable procedural generation, where the game’s world is created on the fly based on a set of rules. This approach allows for infinite and unique worlds, offering endless exploration possibilities for players.
- Customization and Modding: By providing access to world generation algorithms, developers empower modders and content creators to customize and expand the game’s terrain. This fosters a vibrant community around the game, extending its longevity and replayability.
- Performance Optimization: Efficient world generation algorithms, such as perlin noise, contribute to the overall performance of the game. By optimizing the terrain generation process, developers can reduce loading times and ensure a smooth and immersive gameplay experience.
Through the implementation of world generation algorithms like perlin noise, voxel games in C can offer dynamic, visually stunning, and endlessly explorable worlds that captivate players and foster creativity within the gaming community.
Player Character
In the realm of voxel game development, the player character serves as the central figure through which players interact with the game world. Creating a well-defined player character model and implementing intuitive control mechanisms are essential aspects of crafting a compelling and engaging gaming experience.
The player character model not only represents the player’s visual identity within the game but also serves as a foundation for animations, interactions, and gameplay mechanics. Developers must consider factors such as the character’s size, shape, and overall design to ensure it aligns with the game’s aesthetic and gameplay style. A well-crafted player character model can enhance immersion and strengthen the player’s connection to the game.
Equally important are the control mechanisms that govern the player character’s movement and actions. Responsive and intuitive controls empower players to navigate the game world seamlessly and execute actions with precision. Developers must strike a balance between complexity and accessibility, creating control schemes that are both versatile and easy to learn.
The interplay between player character model and control mechanisms is crucial for delivering a satisfying gameplay experience. A well-designed character model that is hindered by clunky controls can frustrate players, while a responsive control scheme paired with a poorly designed character model can limit the player’s immersion and enjoyment.
By understanding the importance of creating a robust player character and implementing intuitive control mechanisms, developers can lay the foundation for an engaging and memorable voxel game that captivates players and keeps them coming back for more.
Gameplay Elements
Gameplay elements are the building blocks of any voxel game, adding depth, challenge, and entertainment to the player’s experience. Enemies, items, and puzzles are essential components that work together to create a dynamic and engaging game world.
Enemies provide conflict and challenge, driving the player’s progression and creating a sense of urgency or danger. They can range from simple AI-controlled mobs to complex boss battles, each requiring unique strategies to defeat. Items serve as rewards or tools, enhancing the player’s abilities or providing access to new areas. They can include weapons, armor, power-ups, or collectible objects that contribute to the player’s growth and customization.
Puzzles introduce mental challenges that require problem-solving skills and logical thinking. They can take various forms, such as environmental puzzles that require manipulation of the game world or logic puzzles that test the player’s deductive reasoning. Puzzles add variety to the gameplay, encourage exploration, and provide a sense of accomplishment upon completion.
The interplay of enemies, items, and puzzles is crucial for creating a balanced and engaging voxel game. Enemies provide obstacles that test the player’s skills and abilities, while items and puzzles offer opportunities for growth and reward. Together, these elements create a dynamic and immersive game world that keeps players engaged and entertained.
In the context of “how to make a voxel game in C,” understanding the significance of gameplay elements is essential for designing and implementing a compelling and enjoyable game. Developers must carefully consider the types of enemies, items, and puzzles that will fit the game’s theme, setting, and target audience. By incorporating these elements effectively, developers can create a voxel game that offers a rich and rewarding gameplay experience.
Physics Engine
In the realm of voxel game development, integrating a physics engine plays a crucial role in enhancing the realism and immersion of the game world. A physics engine simulates the physical properties and interactions of objects, allowing for realistic movement, collisions, and responses to external forces.
Consider a voxel game where the player can interact with objects in the environment, such as pushing blocks, destroying structures, or navigating through complex terrains. Without a physics engine, these interactions would lack the natural feel and realism that players expect. The physics engine calculates the forces, momentum, and collisions between objects, ensuring that they behave in a believable and predictable manner.
The practical significance of using a physics engine in voxel games extends beyond visual aesthetics. It affects gameplay mechanics, puzzle design, and the overall player experience. For instance, a well-implemented physics engine allows players to manipulate objects in creative ways, solve puzzles that involve physical interactions, and engage in dynamic combat scenarios.
Integrating a physics engine into a voxel game in C requires careful consideration of the game’s design and performance requirements. Developers must select an appropriate physics engine that aligns with the game’s physics needs and computational constraints. Common choices include open-source engines like Bullet Physics and commercial engines like Havok Physics. By understanding the importance and practical applications of physics engines, developers can create voxel games that offer immersive and realistic gameplay experiences.
Lighting and Shading
In the realm of voxel game development, lighting and shading play a pivotal role in shaping the visual aesthetics, depth perception, and overall atmosphere of the game world. Effective implementation of these techniques can elevate the player’s experience, making the game more immersive and visually appealing.
-
Dynamic Lighting:
Dynamic lighting allows light sources within the game world to cast real-time shadows and illuminate objects dynamically. This technique mimics natural light behavior, enhancing the realism and immersion of the environment. It enables players to strategically use light and shadow to their advantage, creating stealth-based gameplay elements or puzzles. -
Ambient Occlusion:
Ambient occlusion simulates the indirect lighting that occurs in the absence of direct light sources. It adds depth and realism to the game world by darkening areas where light is obstructed, such as crevices, corners, and the undersides of objects. This technique helps create a more natural and believable visual experience. -
Specular Highlights:
Specular highlights represent the shiny, reflective surfaces of objects. They add a layer of detail and realism to the game’s visuals, making objects appear more metallic or polished. Specular highlights contribute to the perception of depth and enhance the overall aesthetic appeal of the game world. -
Normal Mapping:
Normal mapping is a technique used to add detail and depth to surfaces without increasing the polygon count. It utilizes a special texture map to simulate the surface normals of an object, creating the illusion of finer details and textures. This technique is particularly useful in voxel games, where the blocky nature of voxels can benefit from the added visual complexity.
Through the implementation of lighting and shading techniques, developers can create visually stunning voxel games that captivate players and enhance their immersion in the game world. These techniques add depth, realism, and aesthetic appeal, contributing to a more engaging and memorable gameplay experience.
Optimization
In the context of voxel game development, optimization is paramount to ensure a smooth and enjoyable gameplay experience, especially for large and complex game worlds. Optimization techniques like culling and level of detail play a crucial role in enhancing the game’s performance without compromising visual quality.
Culling is a technique used to selectively render objects based on their visibility to the player. Objects that are outside the player’s field of view or are occluded by other objects can be culled, reducing the number of objects that need to be processed and rendered. This optimization technique can significantly improve the game’s frame rate, especially in scenes with a large number of objects.
Level of detail (LOD) is another important optimization technique used in voxel games. LOD involves using different levels of detail for objects based on their distance from the player. Objects that are closer to the player are rendered with a higher level of detail, while objects that are farther away are rendered with a lower level of detail. This technique reduces the overall processing and rendering cost, allowing for a smoother gameplay experience, particularly in large open-world voxel games.
The practical significance of understanding optimization techniques like culling and level of detail in “how to make a voxel game in C” lies in the ability to create performant and visually appealing voxel games. By implementing these techniques, developers can ensure that their games run smoothly, even on less powerful hardware, and provide a more immersive and enjoyable experience for players.
In summary, optimization techniques like culling and level of detail are essential components of voxel game development in C. Understanding and implementing these techniques empowers developers to create performant and visually stunning voxel games that can captivate players and provide a seamless gaming experience.
Networking
In the realm of voxel game development, networking plays a pivotal role in enabling multiplayer functionality, allowing players to connect and interact with each other in a shared virtual world. Implementing networking protocols is essential for establishing communication channels and synchronizing game states among multiple clients.
-
Client-Server Architecture:
Voxel games often employ a client-server architecture, where a central server manages the game state and facilitates communication between clients. Players connect to the server as clients, sending input commands and receiving updates on the game world. -
Network Protocols:
Networking protocols define the rules and procedures for data exchange over a network. Common protocols used in voxel games include TCP for reliable data transfer and UDP for faster but less reliable communication. -
Data Synchronization:
Maintaining consistency across multiple clients is crucial in multiplayer voxel games. Networking protocols enable the synchronization of game objects, player positions, and other relevant data, ensuring that all players experience a cohesive and seamless gameplay experience. -
Latency Optimization:
Latency, the delay in data transmission, can significantly impact the gameplay experience in multiplayer voxel games. Implementing techniques like interpolation and prediction can minimize the effects of latency, reducing lag and ensuring smooth and responsive gameplay.
Understanding networking and implementing networking protocols in “how to make a voxel game in c” is essential for creating engaging and immersive multiplayer experiences. By establishing reliable communication channels and synchronizing game states, developers can enable players to connect, collaborate, and compete in a shared voxel world.
Voxel games, characterized by their distinct blocky aesthetics, have gained immense popularity in the gaming industry. These games offer a unique blend of creativity and problem-solving, captivating players worldwide. Creating a voxel game in C can be a fulfilling and rewarding experience, and this comprehensive guide will provide you with the essential knowledge and steps to embark on this exciting journey.
The significance of voxel games extends beyond their captivating visuals. They empower players to shape and interact with the game world like never before. From constructing elaborate structures to engaging in dynamic battles, voxel games offer endless possibilities for exploration and creativity. Moreover, the use of C as a programming language provides a robust foundation for developing performant and visually stunning voxel games.
As you delve into the following sections, you will gain insights into the fundamentals of voxel game development in C, including:
- Understanding the core concepts of voxel-based game design
- Mastering essential algorithms and data structures for voxel manipulation
- Implementing lighting and shading techniques to enhance visual fidelity
- Exploring advanced topics such as physics simulation and networking
FAQs on Creating Voxel Games in C
This section provides answers to frequently asked questions (FAQs) related to developing voxel games in C, addressing common concerns and misconceptions.
Question 1: What are the key benefits of using C for voxel game development?
C offers several advantages for voxel game development, including its efficiency, low-level control, and wide range of libraries and tools. It provides direct access to hardware resources, enabling developers to optimize performance and create visually stunning games.
Question 2: What are the fundamental data structures and algorithms used in voxel game development?
Voxel games heavily rely on data structures such as 3D arrays or octrees to represent and manipulate voxel data. Algorithms for tasks like raycasting, collision detection, and terrain generation are essential for creating dynamic and interactive voxel worlds.
Question 3: How can I implement lighting and shading to enhance the visual quality of my voxel game?
Lighting and shading techniques, such as Phong shading and normal mapping, can significantly improve the visual fidelity of voxel games. Understanding these techniques and their implementation in C is crucial for creating immersive and realistic game environments.
Question 4: What are some advanced topics to consider for more sophisticated voxel games?
Advanced topics like physics simulation and networking can add depth and complexity to voxel games. Physics simulation allows for realistic interactions between objects, while networking enables multiplayer functionality, expanding the possibilities for collaboration and competition.
Question 5: Are there any specific challenges or limitations to voxel game development in C?
Voxel game development in C comes with certain challenges, such as managing large voxel datasets and optimizing performance for complex scenes. Understanding these challenges and implementing efficient solutions is essential for creating polished and enjoyable games.
Question 6: Where can I find resources and support for voxel game development in C?
Numerous resources are available online, including tutorials, forums, and open-source projects dedicated to voxel game development in C. Engaging with the community and exploring these resources can provide valuable insights and support throughout the development journey.
By addressing these frequently asked questions, we hope to clarify common misconceptions and provide a foundation for successful voxel game development in C. Remember to continuously learn, experiment, and seek feedback to refine your skills and create captivating voxel experiences.
Transitioning to the next article section…
Conclusion
The journey of creating voxel games in C involves a blend of creativity, technical proficiency, and an understanding of the unique challenges and opportunities that voxel-based game development presents. Throughout this article, we have explored essential concepts, algorithms, and techniques to equip you with the knowledge and skills necessary to embark on this exciting adventure.
Voxel games, with their distinct aesthetics and immersive gameplay, offer limitless possibilities for innovation and player engagement. By embracing the power of C and leveraging the resources and support available, you can bring your voxel game visions to life. Remember to continuously learn, experiment, and seek feedback to refine your craft and create voxel experiences that captivate and inspire players worldwide.
As youof voxel game development in C, we encourage you to embrace the challenges, explore the possibilities, and share your creations with the world. The journey of a thousand voxels begins with a single step, and with dedication and passion, you can build extraordinary voxel worlds that leave a lasting impression on players.
Youtube Video:
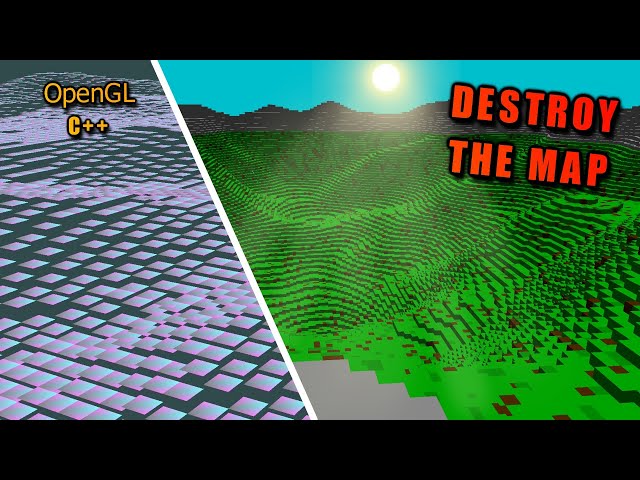