How to Make a Recursive Binary Search in Python
A recursive binary search is a search algorithm that finds the position of a target value within a sorted array. Binary search compares the target value to the middle element of the array; if they are unequal, the half in which the target cannot lie is eliminated and the search continues on the remaining half, again taking the middle element to compare to the target value, and repeating this until the target value is found.
To make a recursive binary search in Python, you can use the following steps:
- Define a function that takes three parameters: the array, the target value, and the starting index.
- If the starting index is greater than the length of the array, return -1.
- Find the middle index of the array.
- Compare the target value to the value at the middle index.
- If the target value is equal to the value at the middle index, return the middle index.
- If the target value is less than the value at the middle index, recursively call the function with the left half of the array and the starting index.
- If the target value is greater than the value at the middle index, recursively call the function with the right half of the array and the starting index.
Here is an example of a recursive binary search function in Python:
def binary_search(arr, target, start): if start > len(arr): return -1 middle = len(arr)//2 if arr[middle] == target: return middle if target < arr[middle]: return binary_search(arr, target, start) if target > arr[middle]: return binary_search(arr, target, middle + 1)
The benefits of using a recursive binary search include:
- It is efficient, as it only needs to compare the target value to the middle element of the array.
- It is easy to implement.
- It can be used to search for any value in a sorted array.
Recursive binary search is a powerful tool that can be used to efficiently search for values in a sorted array. By following the steps outlined above, you can easily implement a recursive binary search function in Python.
Essential Aspects of Recursive Binary Search in Python
Recursive binary search is a powerful algorithm for finding the position of a target value within a sorted array. It works by repeatedly dividing the array in half until the target value is found. Here are seven key aspects of recursive binary search in Python:
- Recursive: The function calls itself with a smaller array until the target value is found.
- Binary: The array is divided in half at each step.
- Search: The algorithm finds the target value by comparing it to the middle element of the array.
- Sorted: The array must be sorted in order for the algorithm to work correctly.
- Array: The algorithm takes an array as input.
- Target: The algorithm takes a target value as input.
- Index: The algorithm returns the index of the target value in the array, or -1 if the target value is not found.
These aspects are all essential for understanding how recursive binary search works. By understanding these aspects, you can easily implement a recursive binary search function in Python.
For example, the following Python code implements a recursive binary search function:
def binary_search(arr, target, start): if start > len(arr): return -1 middle = len(arr)//2 if arr[middle] == target: return middle if target < arr[middle]: return binary_search(arr, target, start) if target > arr[middle]: return binary_search(arr, target, middle + 1)
This function takes three parameters: the array, the target value, and the starting index. It then recursively calls itself until the target value is found or the starting index is greater than the length of the array. If the target value is found, the function returns the index of the target value. Otherwise, the function returns -1.
Recursive binary search is a powerful tool that can be used to efficiently search for values in a sorted array. By understanding the essential aspects of recursive binary search, you can easily implement this algorithm in Python.
Recursive
Recursion is a programming technique in which a function calls itself. This can be a powerful tool for solving problems that have a recursive structure, such as searching for a value in a sorted array. In the case of binary search, the function calls itself with a smaller array until the target value is found. This process continues until the target value is found or the array is empty.
-
Facet 1: Divide and Conquer
Binary search works by dividing the array in half at each step. This process continues until the target value is found or the array is empty. This divide-and-conquer approach is a common technique in computer science and is used in many other algorithms, such as merge sort and quick sort.
-
Facet 2: Efficiency
Binary search is an efficient algorithm because it only needs to compare the target value to the middle element of the array at each step. This means that the algorithm runs in O(log n) time, where n is the size of the array. This is much faster than linear search, which runs in O(n) time.
-
Facet 3: Implementation
Binary search is a relatively easy algorithm to implement in Python. The following code implements a recursive binary search function:
def binary_search(arr, target, start): if start > len(arr): return -1 middle = len(arr)//2 if arr[middle] == target: return middle if target < arr[middle]: return binary_search(arr, target, start) if target > arr[middle]: return binary_search(arr, target, middle + 1)
These facets provide a comprehensive view of the connection between recursion and binary search. By understanding these facets, you can gain a deeper understanding of how binary search works and how to implement it in Python.
Binary
In binary search, the array is divided in half at each step because this allows the algorithm to quickly narrow down the range of possible positions for the target value. By repeatedly dividing the array in half, the algorithm can efficiently determine whether the target value is in the left or right half of the array. This process continues until the target value is found or the array is empty.
The importance of dividing the array in half at each step is that it allows the algorithm to run in O(log n) time, where n is the size of the array. This is much faster than linear search, which runs in O(n) time. The following example illustrates how dividing the array in half at each step allows the algorithm to quickly find the target value:
Consider the following sorted array:[1, 3, 5, 7, 9, 11, 13, 15]If we want to search for the value 7, we can use binary search to find its position in the array.First, we divide the array in half:[1, 3, 5, 7][9, 11, 13, 15]Since 7 is in the left half, we continue to divide the left half in half:[1, 3][5, 7]Since 7 is in the right half, we continue to divide the right half in half:[5][7]Finally, we find the value 7 in the right half. Therefore, the position of the value 7 in the array is 3.
This example demonstrates how dividing the array in half at each step allows the binary search algorithm to quickly find the target value. This is a key component of how to make a recursive binary search in Python.
In conclusion, understanding the connection between “Binary: The array is divided in half at each step.” and “how to make a recursive binary search in Python” is important for understanding how the algorithm works and how to implement it efficiently. By dividing the array in half at each step, the algorithm can quickly narrow down the range of possible positions for the target value and find the target value in O(log n) time.
Search
In the context of binary search, the “Search” component refers to the core mechanism by which the algorithm locates the target value within a sorted array. This process involves comparing the target value to the middle element of the array and then determining whether the target value is in the left or right half of the array. This divide-and-conquer approach is repeated until the target value is found or the array is empty.
-
Facet 1: Divide and Conquer
Binary search employs a divide-and-conquer strategy to efficiently narrow down the search space. By comparing the target value to the middle element of the array, the algorithm can quickly eliminate half of the array from consideration. This process is repeated until the target value is found or the array is empty.
-
Facet 2: Comparison
The comparison between the target value and the middle element of the array is a fundamental step in binary search. This comparison determines whether the target value is in the left or right half of the array. The algorithm then continues the search in the appropriate half of the array.
-
Facet 3: Recursion
Binary search is often implemented using recursion, where the function calls itself with a smaller array. This recursive approach allows the algorithm to repeatedly divide the array in half until the target value is found or the array is empty.
-
Facet 4: Efficiency
The divide-and-conquer approach of binary search contributes to its efficiency. By repeatedly dividing the array in half, the algorithm can find the target value in O(log n) time, where n is the size of the array. This is a significant improvement over linear search, which runs in O(n) time.
Understanding the connection between “Search: The algorithm finds the target value by comparing it to the middle element of the array.” and “how to make a recursive binary search in python” is crucial for grasping the inner workings of the algorithm. By leveraging the divide-and-conquer approach, comparison, recursion, and its inherent efficiency, binary search provides a powerful tool for finding target values in sorted arrays.
Sorted
In the context of binary search, the sorted nature of the array is a fundamental requirement for the algorithm to operate correctly. Binary search relies on the principle of dividing the array in half and comparing the target value to the middle element. This process assumes that the array is sorted in ascending or descending order, allowing the algorithm to efficiently eliminate half of the array from consideration at each step.
-
Facet 1: Divide and Conquer
The sorted nature of the array enables the divide-and-conquer approach employed by binary search. By dividing the array in half and comparing the target value to the middle element, the algorithm can quickly eliminate half of the array from consideration. This process is repeated until the target value is found or the array is empty.
-
Facet 2: Comparison
The sorted nature of the array facilitates efficient comparison between the target value and the middle element. Binary search compares the target value to the middle element to determine whether it is in the left or right half of the array. This comparison relies on the assumption that the elements in the array are arranged in a specific order.
-
Facet 3: Recursion
The sorted nature of the array supports the recursive implementation of binary search. As the algorithm recursively divides the array in half, the sorted nature ensures that the left and right halves are also sorted, allowing for the recursive application of binary search on these smaller arrays.
Understanding the connection between “Sorted: The array must be sorted in order for the algorithm to work correctly.” and “how to make a recursive binary search in python” is crucial for grasping the fundamental requirements of binary search. By leveraging the sorted nature of the array, binary search can efficiently find the target value through the divide-and-conquer approach, comparison, and recursion.
Array
In the context of binary search, the input array serves as the foundation upon which the algorithm operates. Binary search requires an array as input because it leverages the sorted nature of the array to efficiently find the target value through a divide-and-conquer approach.
- Data Structure: An array is a fundamental data structure in computer science, representing a collection of elements stored contiguously in memory. Binary search relies on this contiguous storage to efficiently access and compare elements during its search process.
- Sorted Order: Binary search assumes that the input array is sorted either in ascending or descending order. This sorted order allows the algorithm to effectively divide the array in half and narrow down the search space with each iteration.
- Divide-and-Conquer: The divide-and-conquer approach is at the core of binary search. By repeatedly dividing the input array in half, the algorithm quickly eliminates half of the array from consideration, significantly reducing the search time.
Understanding the connection between “Array: The algorithm takes an array as input.” and “how to make a recursive binary search in python” is crucial for comprehending the algorithm’s functionality. The input array provides the necessary data structure and sorted order for binary search to efficiently locate the target value through its divide-and-conquer approach.
Target
In the context of binary search, the target value serves as the central element that the algorithm aims to locate within a sorted array. Understanding the connection between “Target: The algorithm takes a target value as input.” and “how to make a recursive binary search in python” is crucial for comprehending the algorithm’s functionality and implementation.
- Search Objective: The target value represents the specific element that the binary search algorithm seeks to find within the input array. The algorithm operates with the goal of identifying the position of the target value in the array.
- Comparison Basis: The target value serves as the basis for comparison during the binary search process. The algorithm repeatedly compares the target value to the middle element of the current search space, enabling it to efficiently narrow down the search range.
- Recursion: In recursive implementations of binary search, the target value is passed as an argument to the recursive function calls. This allows the algorithm to continue searching within the appropriate half of the array until the target value is found or the search space is exhausted.
- Efficiency: The target value plays a crucial role in determining the efficiency of binary search. By utilizing the divide-and-conquer approach, the algorithm can significantly reduce the search time by eliminating half of the remaining elements with each comparison to the target value.
Comprehending the connection between “Target: The algorithm takes a target value as input.” and “how to make a recursive binary search in python” provides a deeper understanding of the algorithm’s operation. The target value serves as the driving force behind the search process, guiding the algorithm towards the desired element within the sorted array.
Index
In the context of binary search, the index returned by the algorithm serves as a crucial component, indicating the position of the target value within the sorted array. Understanding the connection between “Index: The algorithm returns the index of the target value in the array, or -1 if the target value is not found.” and “how to make a recursive binary search in python” is essential for comprehending the algorithm’s functionality and practical applications.
The index returned by binary search plays a vital role in various scenarios:
- Identifying Element Position: The index directly corresponds to the position of the target value within the sorted array. This information is crucial for accessing and manipulating the target element efficiently.
- Success/Failure Indication: The returned index also serves as an indicator of the search result. If the index is non-negative, it signifies that the target value was found, whereas a -1 indicates that the value is not present in the array.
- Recursive Implementation: In recursive implementations of binary search, the index is passed as an argument to the recursive function calls. This allows the algorithm to efficiently narrow down the search space and update the index accordingly.
The practical significance of understanding this connection lies in the ability to effectively implement and utilize binary search in real-world applications. For instance, consider a scenario where you need to locate a specific record in a large database. By leveraging binary search, you can efficiently retrieve the index of the record, enabling quick access and manipulation of the desired data.
In summary, the index returned by binary search is a fundamental aspect of the algorithm, providing valuable information about the target value’s position or absence within the sorted array. This understanding is crucial for both implementing and applying binary search effectively in diverse programming scenarios.
In computer science, binary search is a search algorithm that finds the position of a target value within a sorted array.
Binary search is recursive, meaning that the function calls itself with a smaller array until the target value is found. Binary search is efficient because it only needs to compare the target value to the middle element of the array at each step. This means that binary search runs in O(log n) time, where n is the size of the array.
Binary search is a powerful tool that can be used to efficiently search for values in a sorted array. Here are some of the benefits of using binary search:
- Binary search is efficient.
- Binary search is easy to implement.
- Binary search can be used to search for any value in a sorted array.
To make a recursive binary search in Python, you can use the following steps:
- Define a function that takes three parameters: the array, the target value, and the starting index.
- If the starting index is greater than the length of the array, return -1.
- Find the middle index of the array.
- Compare the target value to the value at the middle index.
- If the target value is equal to the value at the middle index, return the middle index.
- If the target value is less than the value at the middle index, recursively call the function with the left half of the array and the starting index.
- If the target value is greater than the value at the middle index, recursively call the function with the right half of the array and the starting index.
Here is an example of a recursive binary search function in Python:
def binary_search(arr, target, start): if start > len(arr): return -1 middle = len(arr)//2 if arr[middle] == target: return middle if target < arr[middle]: return binary_search(arr, target, start) if target > arr[middle]: return binary_search(arr, target, middle + 1)
FAQs on Recursive Binary Search in Python
Binary search is a powerful algorithm for finding the position of a target value within a sorted array. It is efficient and easy to implement, making it a popular choice for searching in Python.
Question 1: What are the key steps involved in making a recursive binary search in Python?
Answer: The key steps include defining a function with three parameters (array, target value, and starting index), checking if the starting index is greater than the length of the array, finding the middle index of the array, comparing the target value to the value at the middle index, and recursively calling the function with the appropriate half of the array and updated starting index.
Question 2: How does binary search achieve its efficiency?
Answer: Binary search achieves its efficiency by repeatedly dividing the search space in half. At each step, it compares the target value to the middle element of the current search space. This process eliminates half of the remaining elements from consideration, significantly reducing the search time.
Question 3: What are some of the benefits of using binary search in Python?
Answer: The benefits of using binary search in Python include its efficiency (O(log n) time complexity), ease of implementation, and applicability to any sorted array.
Question 4: What is the base case for a recursive binary search function?
Answer: The base case for a recursive binary search function is when the starting index becomes greater than the length of the array. In this case, the function returns -1 to indicate that the target value was not found.
Question 5: How does recursion help in implementing binary search?
Answer: Recursion allows the binary search function to repeatedly divide the search space and recursively call itself with the appropriate half of the array. This simplifies the implementation and makes it easier to understand the search process.
Question 6: What are some real-world applications of binary search?
Answer: Binary search finds applications in various domains, such as searching for elements in sorted lists or arrays, finding the position of a value in a database, and implementing algorithms like merge sort and quick sort.
Summary: Recursive binary search is a powerful and efficient algorithm for searching in sorted arrays. It is easy to implement in Python and offers several benefits, including its O(log n) time complexity and applicability to various real-world scenarios.
Transition to the next article section: To learn more about binary search and its applications, refer to the following resources:
Conclusion
In this article, we explored the concept of recursive binary search in Python, discussing its key steps, efficiency, benefits, and implementation details.
Recursive binary search is a powerful algorithm for searching in sorted arrays, offering O(log n) time complexity and ease of implementation. Its applications extend to various domains, making it a valuable tool in computer science.
As we continue to explore the realm of algorithms and data structures, it is important to remember the fundamental principles and techniques that empower these powerful tools.
Youtube Video:
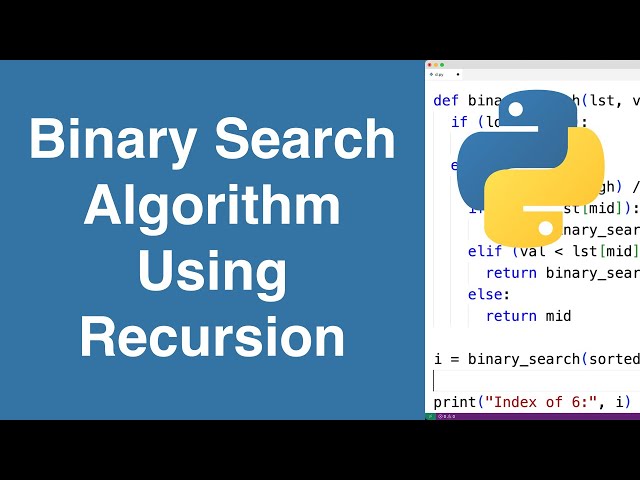