How to Make Stored Data to HTML JavaScript
Storing data in HTML JavaScript is a common task in web development. Data can be stored in a variety of formats, such as arrays, objects, and JSON. Once data is stored, it can be used to populate web pages, create interactive applications, and perform other tasks.
There are a number of different ways to store data in HTML JavaScript. However, the most common methods are:
- Arrays: Arrays are used to store a collection of values. Each value in an array is assigned an index, which can be used to access the value.
- Objects: Objects are used to store a collection of key-value pairs. Each key is associated with a value, which can be any type of data.
- JSON: JSON (JavaScript Object Notation) is a text-based data format that is used to represent objects and data structures. JSON data can be stored in variables, passed between functions, and sent over the network.
Once data is stored in HTML JavaScript, it can be used to populate web pages, create interactive applications, and perform other tasks. For example, data can be used to:
- Create dynamic content that changes based on user input.
- Store user preferences and settings.
- Track user activity and behavior.
- Perform calculations and other data processing tasks.
Storing data in HTML JavaScript is a powerful way to improve the functionality and usability of your web pages. By following the steps outlined in this article, you can learn how to store data in HTML JavaScript and use it to improve your web pages.
How to Make Stored Data to HTML JavaScript
Storing data in HTML JavaScript is a common task in web development. However, it can be a complex task, especially if you are new to programming. In this article, we will discuss the essential aspects of storing data in HTML JavaScript.
- Data Types: The first step is to understand the different data types that can be stored in HTML JavaScript. These include strings, numbers, booleans, arrays, and objects.
- Variables: Variables are used to store data in HTML JavaScript. Variables are declared using the var keyword, followed by the variable name.
- Arrays: Arrays are used to store a collection of values. Arrays are declared using the [] notation, and each value in an array is assigned an index.
- Objects: Objects are used to store a collection of key-value pairs. Objects are declared using the {} notation, and each key is associated with a value.
- JSON: JSON (JavaScript Object Notation) is a text-based data format that is used to represent objects and data structures. JSON data can be stored in variables, passed between functions, and sent over the network.
- Storage: Data can be stored in HTML JavaScript using a variety of storage mechanisms, such as cookies, local storage, and session storage.
- Security: It is important to consider the security of your data when storing it in HTML JavaScript. Data should be encrypted and stored in a secure location.
These are just a few of the essential aspects of storing data in HTML JavaScript. By understanding these concepts, you can improve the functionality and usability of your web pages.
Data Types
Understanding data types is crucial for storing data in HTML JavaScript because it determines how data is represented, stored, and manipulated. Different data types have different properties and methods, and choosing the appropriate data type for a specific purpose is essential for efficient and effective data management.
For example, strings are used to store text data, numbers are used to store numeric data, and booleans are used to store true or false values. Arrays are used to store a collection of values, and objects are used to store a collection of key-value pairs. By understanding the properties and methods of each data type, developers can store and manipulate data in a way that is tailored to the specific needs of their application.
In summary, understanding data types is a fundamental aspect of storing data in HTML JavaScript. By choosing the appropriate data type for a specific purpose, developers can ensure that data is stored and manipulated in an efficient and effective manner.
Variables
Variables play a crucial role in storing data in HTML JavaScript, as they provide a way to associate a name with a specific value. This allows data to be easily accessed, manipulated, and modified throughout the code.
- Data Storage: Variables serve as containers for data, enabling developers to store and retrieve information as needed. They can store various types of data, such as strings, numbers, arrays, and objects, providing flexibility in data management.
- Data Manipulation: Variables not only store data but also allow for data manipulation. Developers can modify the value of a variable, perform calculations, and update data dynamically, making it possible to create interactive and responsive web applications.
- Code Organization: Variables help organize code by providing meaningful names to data. This improves code readability and maintainability, making it easier for developers to understand the purpose and flow of the code.
- Data Sharing: Variables facilitate data sharing between different parts of a program. By passing variables as parameters to functions or accessing them globally, developers can share data efficiently and reduce the need for redundant data storage.
In summary, variables are fundamental to storing data in HTML JavaScript. They provide a structured and flexible way to manage data, enabling developers to store, manipulate, and share information effectively, ultimately contributing to the creation of robust and maintainable web applications.
Arrays
Arrays are an essential aspect of storing data in HTML JavaScript. They provide a structured way to store and organize data, enabling efficient access and manipulation. Understanding how arrays work is crucial for effectively managing data in web applications.
- Data Organization: Arrays allow developers to group related data items together, making it easier to manage and access data. By assigning each item an index, data can be retrieved or modified quickly and efficiently.
- Efficient Storage: Arrays provide a compact way to store data, as they allocate memory contiguously for all elements. This contiguous storage optimizes memory usage and improves performance, especially when working with large datasets.
- Dynamic Data: Arrays are dynamic in nature, meaning they can grow or shrink as needed. Developers can add or remove elements from arrays at runtime, providing flexibility in data management and adapting to changing requirements.
- Iteration and Processing: Arrays support efficient iteration and processing of data. Developers can use loops to iterate over each element in an array, perform calculations, or manipulate data, simplifying complex data operations.
In summary, arrays are a powerful tool for storing data in HTML JavaScript. They provide efficient storage, organization, and manipulation of data, making them essential for managing complex datasets and building dynamic web applications.
Objects
Objects play a crucial role in “how to make stored data to HTML JavaScript” because they provide a structured and flexible way to store and organize complex data. Objects are particularly useful for representing real-world entities or concepts, where data can be categorized and accessed through meaningful keys.
For example, consider an e-commerce website that needs to store product information. Each product can be represented as an object, with properties such as name, price, description, and images. By using objects, developers can easily store and retrieve product data, making it accessible for display on web pages, shopping cart management, and order processing.
Another advantage of objects is their ability to nest other objects or arrays. This allows for hierarchical data structures, which are useful for representing complex relationships between data items. For instance, in the e-commerce example, each product object could have a nested array of reviews, where each review is represented as an object with its own properties (e.g., author, rating, comment).
In summary, objects are a powerful tool for storing data in HTML JavaScript because they provide a structured and flexible way to organize and access complex data. By representing data as objects, developers can create dynamic and interactive web applications that can efficiently manage and display complex information.
JSON
JSON plays a crucial role in “how to make stored data to HTML JavaScript” because it provides a standardized format for representing complex data. JSON data can be easily converted to and from JavaScript objects, making it an ideal choice for exchanging data between the client and server, or for storing data in a database.
- Data Exchange: JSON is widely used for data exchange between the client and server in web applications. It is a lightweight and efficient format that can be easily parsed and processed by both JavaScript and server-side languages. This makes it an ideal choice for transmitting data between different components of a web application, such as passing data from a form on the client to a server-side script for processing.
- Data Storage: JSON can also be used to store data in a database. It is a flexible format that can represent a wide variety of data structures, making it suitable for storing complex data objects. Additionally, JSON data can be easily indexed and queried, making it efficient for data retrieval.
- Data Sharing: JSON is a common format for sharing data between different applications and services. Its standardized structure makes it easy to integrate with various systems and platforms. This enables developers to share data between different components of their application, or even with third-party applications.
- Data Portability: JSON data is portable across different platforms and programming languages. This makes it easy to move data between different systems or applications, regardless of the underlying technology stack. This portability is particularly useful for applications that need to exchange data with other systems or for data migration purposes.
In summary, JSON is a versatile and powerful data format that plays a crucial role in “how to make stored data to HTML JavaScript”. Its ability to represent complex data structures, facilitate data exchange, and enable data portability makes it an essential tool for building modern web applications.
Storage
Data storage is an essential aspect of “how to make stored data to html javascript”. It enables websites and applications to persist data beyond the current session or page load, allowing users to save preferences, track their progress, and interact with dynamic content.
-
Local Storage
Local storage is a mechanism for storing data on the client-side, specifically within the user’s browser. Data stored in local storage persists even after the browser is closed and reopened. This makes it suitable for storing user preferences, settings, and other data that needs to be retained across sessions.
-
Session Storage
Session storage is similar to local storage, but data stored in session storage is only available during the current browsing session. Once the browser is closed, the data is lost. Session storage is useful for storing temporary data that needs to be accessible throughout the session, such as shopping cart contents or form data.
-
Cookies
Cookies are small text files that are stored on the client-side by the web server. They are used to store information about the user’s browsing activity, such as login credentials, language preferences, and tracking data. Cookies are typically used to maintain state between the client and server, and to personalize the user experience.
The choice of storage mechanism depends on the specific requirements of the application. Local storage is suitable for data that needs to be persistent across sessions, while session storage is ideal for temporary data. Cookies are commonly used for maintaining state and tracking user activity.
Security
Security is a crucial aspect of “how to make stored data to html javascript” because it ensures the protection and privacy of sensitive data. Storing data in HTML JavaScript without proper security measures can expose it to unauthorized access, data breaches, and malicious attacks.
One of the primary threats to data security in HTML JavaScript is cross-site scripting (XSS) attacks. XSS attacks occur when malicious scripts are injected into a web page, allowing attackers to steal sensitive information, such as login credentials and session cookies. To prevent XSS attacks, it is essential to validate and sanitize user input, and to use secure coding practices to prevent the execution of malicious scripts.
Another important security consideration is data encryption. Encrypting data before storing it in HTML JavaScript makes it unreadable to unauthorized users, even if they gain access to the data. Encryption algorithms, such as AES-256, can be used to encrypt data, ensuring its confidentiality.
In addition to encryption, data should also be stored in a secure location. This may involve using a database with access control mechanisms, or storing data on a secure server with limited access.
By implementing proper security measures, developers can protect sensitive data from unauthorized access and ensure the integrity and confidentiality of stored data.
“How to make stored data to HTML JavaScript” refers to the process of saving data in a web browser using HTML JavaScript, enabling it to be accessed and manipulated by web applications. This involves utilizing various data storage mechanisms, such as variables, arrays, objects, and databases, to persistently store data beyond the current session or page load.
Storing data in HTML JavaScript offers numerous benefits. It allows websites and applications to retain user preferences, track user activity, and provide personalized experiences. Additionally, it enables the creation of dynamic and interactive web applications that can store and retrieve data from databases or local storage, making them more efficient and user-friendly.
To delve deeper into “how to make stored data to html javascript,” let’s explore the different data storage mechanisms and techniques used in HTML JavaScript:
FAQs on “How to Make Stored Data to HTML JavaScript”
This section addresses frequently asked questions (FAQs) to provide further clarification on the topic of “how to make stored data to HTML JavaScript”.
Question 1: What are the benefits of storing data in HTML JavaScript?
Answer: Storing data in HTML JavaScript offers several benefits, including the ability to retain user preferences, track user activity, and create dynamic and interactive web applications. It allows websites and applications to provide personalized experiences and efficiently manage data.
Question 2: What are the different data storage mechanisms used in HTML JavaScript?
Answer: HTML JavaScript provides various data storage mechanisms, such as variables, arrays, objects, and databases. Variables are used to store single values, while arrays can store multiple values in an ordered collection. Objects allow for the storage of key-value pairs, and databases enable the storage and management of large amounts of structured data.
Question 3: How can I protect the security of stored data in HTML JavaScript?
Answer: Ensuring the security of stored data is crucial. Implement encryption techniques to protect data confidentiality, and store data in secure locations with restricted access to prevent unauthorized retrieval.
Question 4: What are the best practices for storing data in HTML JavaScript?
Answer: Follow best practices such as using descriptive variable names, organizing data logically, and implementing error handling to ensure the integrity and accessibility of stored data.
Question 5: How can I store data permanently in HTML JavaScript?
Answer: To store data permanently in HTML JavaScript, utilize mechanisms like local storage or indexedDB. These allow data to persist even after the browser session ends, providing long-term storage capabilities.
Question 6: What are the limitations of storing data in HTML JavaScript?
Answer: While HTML JavaScript provides convenient data storage options, there are limitations. Stored data may be vulnerable to security breaches if not properly secured. Additionally, the amount of data that can be stored may be constrained by browser limitations.
Summary: Understanding how to make stored data to HTML JavaScript is essential for creating dynamic and interactive web applications. By leveraging the available data storage mechanisms and adhering to best practices, developers can effectively manage and protect stored data, enhancing the user experience and ensuring the integrity of their applications.
Transition to the next article section: This concludes the FAQs on “how to make stored data to HTML JavaScript”.
Conclusion on “How to Make Stored Data to HTML JavaScript”
In this article, we explored the intricacies of “how to make stored data to HTML JavaScript”. We examined the various data storage mechanisms, including variables, arrays, objects, and databases, and discussed their respective strengths and applications. Additionally, we emphasized the importance of data security and outlined best practices for protecting stored data from unauthorized access and breaches.
Understanding how to effectively store data in HTML JavaScript is paramount for developing robust and interactive web applications. By leveraging the available data storage mechanisms and adhering to best practices, developers can create applications that seamlessly manage and protect stored data, enhance the user experience, and ensure the integrity of their applications. As the web evolves and new technologies emerge, the techniques discussed in this article will continue to serve as a solid foundation for effective data storage in HTML JavaScript.
Youtube Video:
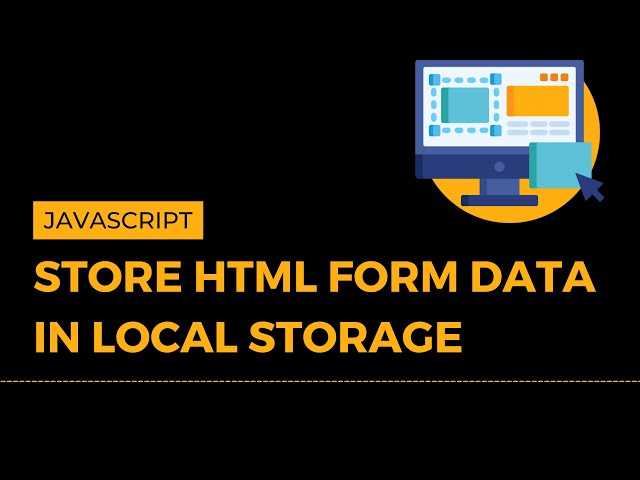