how to make a macro in razor
A macro in Razor is a way to reuse a block of code. This can be useful for reducing the amount of code that you need to write, and it can also help to improve the readability of your code. To create a macro in Razor, you need to define a template with the name of the macro, and then you can use the macro by calling it in your code.
Here are the steps on how to create a macro in Razor:
- Define a template with the name of the macro. The template should contain the code that you want to reuse.
- Call the macro in your code using the @ symbol followed by the name of the macro.
Here is an example of how to create a macro in Razor:
@{// Define a macro called "MyMacro"@macro MyMacro {
}}// Call the macro in your code@MyMacro
This macro can be used to display the text “Hello, world!” in your code. You can call the macro as many times as you want.
Benefits of using macros in Razor:
- Reduced code duplication
- Improved code readability
- Easier maintenance
Macros are a powerful tool that can help you to improve the quality of your Razor code. By following the steps outlined in this article, you can easily create and use macros in your own code.
how to make a macro in razor
Macros are a powerful tool in Razor that can help you to improve the quality of your code. By understanding the essential aspects of macros, you can use them effectively to reduce code duplication, improve readability, and make your code easier to maintain.
- Definition: A macro is a way to reuse a block of code.
- Creation: Macros are created by defining a template with the name of the macro.
- Invocation: Macros are invoked by calling them in your code using the @ symbol followed by the name of the macro.
- Benefits: Macros can reduce code duplication, improve readability, and make your code easier to maintain.
- Example: A simple macro can be used to display the text “Hello, world!” in your code.
- Best Practices: Use macros to avoid code duplication and improve the readability of your code.
Macros are a versatile tool that can be used in a variety of ways to improve your Razor code. By understanding the essential aspects of macros, you can use them effectively to create more efficient, readable, and maintainable code.
Definition
In the context of “how to make a macro in Razor”, this definition serves as the foundation for understanding the concept and purpose of macros. Macros allow developers to create reusable code blocks that can be invoked throughout their Razor codebase, fostering code efficiency and reducing the need for repetitive coding.
- Code Reusability: Macros promote code reusability by encapsulating commonly used code blocks into named templates. This eliminates the need to rewrite the same code multiple times, minimizing redundancy and potential errors.
- Improved Readability: By extracting reusable code into macros, Razor code becomes more readable and maintainable. Macros act as self-documenting units, making it easier for developers to understand the purpose and functionality of specific code sections.
- Simplified Maintenance: Macros simplify code maintenance by centralizing reusable code. When changes are required, developers only need to modify the macro definition, ensuring that all instances of the macro are updated accordingly, reducing the risk of inconsistencies and errors.
- Increased Productivity: Macros enhance developer productivity by reducing the time spent on writing repetitive code. Instead, developers can focus on more complex and value-added tasks, leading to faster development cycles.
Overall, the definition of a macro as a reusable code block is central to understanding how macros empower developers to write efficient, readable, and maintainable Razor code.
Creation
In the context of “how to make a macro in Razor”, understanding the process of macro creation is essential. Macros are defined by creating a template with a unique name. This template serves as the blueprint for the reusable code block, encapsulating the functionality to be reused throughout the Razor codebase.
The significance of macro creation lies in its impact on code reusability and maintainability. By defining a template, developers establish a named entity that can be invoked whenever the encapsulated code is required. This eliminates the need for repetitive coding, reducing the risk of errors and inconsistencies. Additionally, it promotes code readability by organizing reusable code into logical units, making the codebase more structured and easier to navigate.
For instance, consider a Razor application that frequently displays a navigation menu. Instead of duplicating the menu code in multiple views, a macro can be created to encapsulate the menu’s HTML structure and logic. This macro can then be invoked in each view that requires the menu, ensuring consistency and simplifying maintenance. If any changes to the menu are necessary, developers only need to modify the macro template, and all instances of the menu will be updated accordingly.
In summary, understanding the process of macro creation is crucial for harnessing the benefits of code reusability and maintainability in Razor. By defining templates with unique names, developers can create reusable code blocks that enhance the efficiency, readability, and maintainability of their Razor applications.
Invocation
In the context of “how to make a macro in Razor”, understanding macro invocation is crucial for effectively utilizing macros and achieving code reusability. Macros are invoked by calling them in your code using the @ symbol followed by the name of the macro. This invocation mechanism is a fundamental aspect of working with macros in Razor.
The importance of macro invocation lies in its impact on code reusability and maintainability. By invoking macros, developers can reuse predefined code blocks throughout their Razor codebase, eliminating the need for repetitive coding and reducing the risk of errors and inconsistencies. This invocation mechanism promotes code readability and organization, making the codebase more structured and easier to navigate.
For instance, consider a Razor application that requires the display of a copyright notice in multiple views. Instead of duplicating the copyright notice code in each view, a macro can be created to encapsulate the notice’s content and formatting. This macro can then be invoked in each view where the copyright notice is required, ensuring consistency and simplifying maintenance. If any changes to the copyright notice are necessary, developers only need to modify the macro definition, and all instances of the notice will be updated accordingly.
In summary, understanding macro invocation is essential for harnessing the benefits of code reusability and maintainability in Razor. By invoking macros using the @ symbol followed by the macro’s name, developers can effectively reuse predefined code blocks, leading to more efficient, readable, and maintainable Razor applications.
Benefits
In the context of “how to make a macro in Razor”, understanding the benefits of macros is crucial for appreciating their value and effectively utilizing them in your code. Macros offer several key benefits that contribute to the overall quality and maintainability of your Razor codebase.
Reduced Code Duplication: Macros help reduce code duplication by allowing developers to encapsulate reusable code blocks into named templates. This eliminates the need to repeat the same code in multiple places, minimizing redundancy and potential errors. By centralizing reusable code, macros promote a DRY (Don’t Repeat Yourself) approach, leading to cleaner and more maintainable code.
Improved Readability: Macros enhance code readability by organizing reusable code into logical units. Instead of scattering reusable code throughout the codebase, macros gather it into named templates, making it easier for developers to understand the purpose and functionality of specific code sections. This improved readability reduces the cognitive load on developers, making it easier to maintain and extend the codebase.
Easier Maintenance: Macros simplify code maintenance by centralizing reusable code. When changes are required, developers only need to modify the macro definition, ensuring that all instances of the macro are updated accordingly. This centralized maintenance approach reduces the risk of inconsistencies and errors, saving time and effort during the development and maintenance process.
In summary, understanding the benefits of macros in terms of reduced code duplication, improved readability, and easier maintenance is essential for harnessing their full potential in Razor. By leveraging macros effectively, developers can create more efficient, readable, and maintainable Razor applications.
Example
This example showcases the fundamental concept of macro creation and invocation in Razor. By defining a simple macro that displays the text “Hello, world!”, we illustrate how macros can be used to encapsulate reusable code blocks and improve code readability and maintainability.
- Code Reusability: The simple macro serves as a practical example of how macros promote code reusability. Instead of repeating the “Hello, world!” code in multiple places, we can define a macro and invoke it wherever needed, reducing the risk of errors and inconsistencies.
- Improved Readability: The example demonstrates how macros enhance code readability by organizing reusable code into a named template. This makes it easier for developers to understand the purpose and functionality of the “Hello, world!” code, as it is encapsulated in a logical unit.
- Simplified Maintenance: The example highlights the maintenance benefits of macros. If we need to change the “Hello, world!” message, we only need to modify the macro definition, ensuring that all instances of the macro are updated accordingly. This centralized maintenance approach reduces the risk of inconsistencies and errors.
In summary, this example provides a practical illustration of how macros can be used to improve code reusability, readability, and maintainability in Razor applications.
Best Practices
In the context of “how to make a macro in Razor”, understanding best practices is crucial for leveraging macros effectively and maximizing their benefits. One key best practice is to use macros to avoid code duplication and improve the readability of your code.
Code duplication occurs when the same code is repeated in multiple places within a codebase. This can lead to errors and inconsistencies, making it difficult to maintain and extend the codebase. Macros provide a solution to this problem by allowing developers to encapsulate reusable code blocks into named templates. By invoking macros instead of duplicating code, developers can reduce the risk of errors and inconsistencies, leading to a more maintainable and reliable codebase.
Code readability is another important aspect of code quality. Readable code is easy to understand and maintain, reducing the time and effort required for development and maintenance tasks. Macros can improve code readability by organizing reusable code into logical units. Instead of scattering reusable code throughout the codebase, macros gather it into named templates, making it easier for developers to understand the purpose and functionality of specific code sections. This improved readability reduces the cognitive load on developers, making it easier to maintain and extend the codebase.
In summary, understanding and applying the best practice of using macros to avoid code duplication and improve readability is essential for creating high-quality Razor applications. By leveraging macros effectively, developers can write code that is maintainable, reliable, and easy to understand.
In Razor, macros are a powerful tool that allow developers to create reusable code blocks. This can be useful for reducing the amount of code that you need to write, and it can also help improve the readability and maintainability of your code.
To create a macro in Razor, you need to define a template with the name of the macro. The template should contain the code that you want to reuse. You can then call the macro in your code using the @ symbol followed by the name of the macro.
For example, the following macro can be used to display the current date and time:
@macro CurrentDateTime {
The current date and time is @DateTime.Now.
}
You can then call this macro in your code using the following syntax:
@CurrentDateTime
This will output the current date and time to the page.
Macros can be used for a variety of purposes, such as:
- Creating reusable components
- Encapsulating complex logic
- Improving the readability and maintainability of your code
If you are working with Razor, then macros are a valuable tool that you should learn how to use. They can help you write more efficient, readable, and maintainable code.
FAQs about Macros in Razor
Macros are a powerful tool in Razor that can help you to improve the quality of your code. Here are some frequently asked questions about macros in Razor:
Question 1: What are macros?
Macros are a way to reuse a block of code in Razor. They are defined using a template with the name of the macro, and they can be called in your code using the @ symbol followed by the name of the macro.
Question 2: Why should I use macros?
Macros can be used to reduce code duplication, improve readability, and make your code easier to maintain.
Question 3: How do I create a macro?
To create a macro, you need to define a template with the name of the macro. The template should contain the code that you want to reuse.
Question 4: How do I call a macro?
To call a macro, you can use the @ symbol followed by the name of the macro.
Question 5: What are some examples of how I can use macros?
Macros can be used to create reusable components, encapsulate complex logic, and improve the readability and maintainability of your code.
Question 6: Are there any best practices for using macros?
Yes, there are a few best practices for using macros. First, use macros to avoid code duplication. Second, use macros to improve the readability of your code. Third, use macros to encapsulate complex logic.
Macros are a valuable tool that you can use to improve the quality of your Razor code. By understanding how to create and use macros, you can write more efficient, readable, and maintainable code.
Continue reading:
Advanced Techniques for Using Macros in Razor
Conclusion
Macros are a powerful tool that can help you to improve the quality of your Razor code. They can reduce code duplication, improve readability, and make your code easier to maintain. In this article, we have explored how to create and use macros in Razor, and we have discussed some of the best practices for using them.
As you gain more experience with macros, you will find that they are a valuable tool for writing more efficient, readable, and maintainable Razor code. We encourage you to experiment with macros and see how they can benefit your code.
Youtube Video:
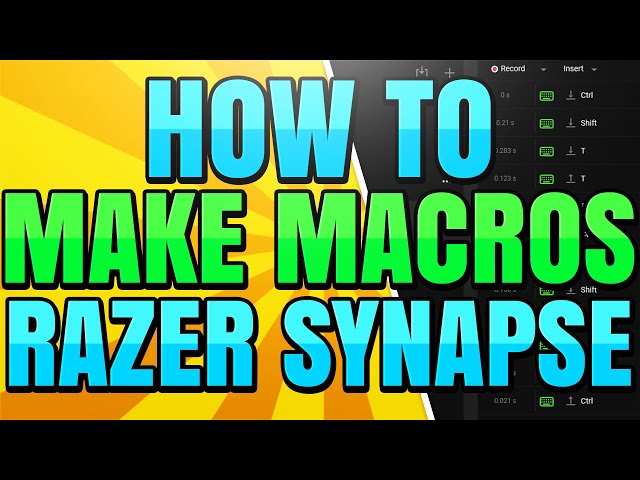